Advanced JUnit Testing Strategies for Robust Java Applications
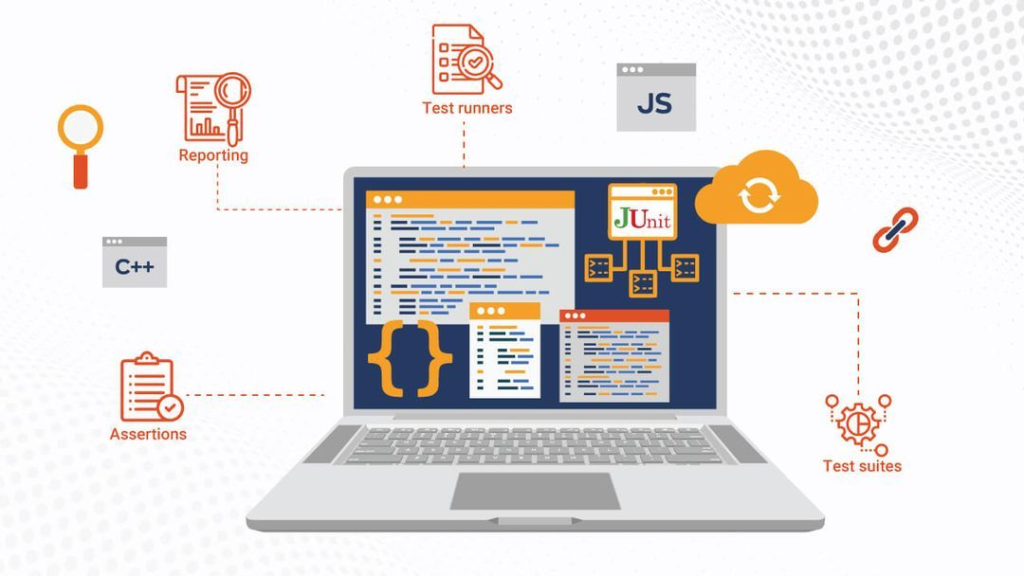
JUnit is a powerful testing framework applied within Java programming to help developers test that things work the way they’re supposed to. Execute JUnit testing to automatically see if your code behaves correctly. If anything goes wrong, JUnit immediately gives you notice. It acts almost like a safety net, catching the errors before they can result in big problems.
In the times of modern development, rapid growth means that testing can no longer be basic. Applications grow in complexity and so do the bugs. That’s why the need for advanced test strategies is urgent. Such methods will help to find those tricky bugs that simple tests won’t be able to reveal. In this way, you can make your applications more reliable and robust, meaning fewer crashes, fewer surprises, and a much smoother experience for your users.
Understanding JUnit Basics
JUnit is perhaps one of the most famous frameworks in the Java developer world. It was created years ago and trusted worldwide by the developers. In a nutshell, JUnit Testing makes it possible for you to write tests which could run themselves. These tests ensure that your code does what it should do. When there is something wrong, JUnit points this out.
It’s a very easy framework to get along with but, at the same time, very powerful too. It comes with great features that make testing a lot easier and more efficiently carried out. Examples include test grouping, the running of tests in order, and even test suites that can cover much larger pieces of your application. Another amazing thing about JUnit is that it shows clear feedback if some of the tests pass but others fail.
Unit testing plays a major role in software development. It allows you to catch errors right from the beginning of your project before tiny mistakes grow to become major headaches. Testing the discrete units of your code assures you that each part is functioning correctly. Therefore, debugging becomes rather easy with no complexity, saving you time and energy in the longer run. The unit tests serve as documentation, too: they show how your code is supposed to work.
Advanced JUnit Testing Strategies
As your Java applications grow, so does the need for more advanced testing strategies. Simple tests might catch basic errors, but what about the tricky bugs? That’s where advanced strategies come in. They help you test more thoroughly, catching the issues that basic tests might miss. Let’s explore some of these strategies.
Parameterized Tests
Parameterized tests are a powerful feature in JUnit. They allow you to run the same test with different inputs. This is useful when you want to test a method with various data sets. Instead of writing separate tests for each input, you write one test and provide the inputs as parameters. JUnit runs the test for each set of parameters, checking if the method behaves correctly.
For example, imagine you have a method that adds two numbers. You want to test it with different pairs of numbers. Instead of writing a separate test for each pair, you write one parameterized test. You then provide the pairs of numbers as parameters. JUnit runs the test for each pair, making sure the method returns the correct sum.
The benefits of parameterized tests are clear. They save you time by reducing the number of tests you need to write. They also make your tests easier to maintain. If you need to change the test, you only have to change it in one place. Parameterized tests also make it easier to spot patterns. By running the same test with different inputs, you can see how your method behaves under different conditions.
Test Fixtures and Suite Setup
Test fixtures are another important feature in JUnit. They allow you to set up a common environment for your tests. This is useful when you have multiple tests that need the same setup. Instead of repeating the setup code in each test, you put it in a fixture. JUnit runs the fixture before each test, ensuring the environment is ready.
JUnit has provided several setup annotations. The @BeforeClass and @AfterClass hooks once run before and after all the tests in a class, respectively. Use it to set up and tear down resources shared by all tests, such as databases or file systems. There is creation of @Before and @After hooks, running before and after each test, accordingly. Use them for the test-specific setup and takedown, like mock objects or temporary files.
Using test fixtures offers several advantages. Mainly, the setup code is moved to one place, lessening code duplication. Your tests become easier to maintain: you would need to change the set up in only one place when changes are needed. Implementing fixtures makes your tests more reliable, because they ensure the environment is always set up correctly, removing many opportunities for errors due to wrong setup.
Mocking and Stubbing with JUnit
Mocking and stubbing stand out for being very important techniques within unit tests. They allow a developer to test their code in isolation without actually connecting to other systems. For example, a developer might be required to test a method that talks to a database. Rather than use an actual database-which is often sluggish and temperamental-a mock is used in its place. This mock will act exactly like the real database but is much quicker and far easier to control.
Mockito is one of the most well-known libraries to make mock objects in Java. Mockito works well with JUnit, and hence creating mock objects is pretty straightforward. Mockito allows you to create mock objects, specify behavior for these mock objects, and then verify interactions. Example usage: You can create a mock database, specify that it should return value A when queried for “something”, and then verify that “something” was queried.
Stubbing is similar to mocking but is all about returning canned responses. For example, you might want to test a method that calls some web service. Instead of calling the real service-who knows how slow or unreliable it might be-you use a stub. The stub returns a pre-canned response, and you can test how your method handles it.
Mocking and stubbing have many benefits. They make your tests faster by eliminating the need to interact with slow or unreliable systems. They also make your tests more reliable by removing external dependencies. By testing your code in isolation, you can focus on the code itself, making it easier to spot and fix bugs.
Custom JUnit Rules
JUnit rules are a powerful feature that allows you to add additional behavior to your tests. Custom rules are especially useful when you need to apply the same behavior to multiple tests. Instead of repeating the behavior in each test, you create a custom rule. The rule is applied automatically to all the tests in a class, reducing code duplication and improving maintainability.
Creating a custom rule is straightforward. You create a class that implements the TestRule interface, and override its apply method. In the apply method, you specify the behavior you want to add. For example, you might create a custom rule that logs the start and end of each test. The rule would log a message at the start of the test, run the test, and log another message at the end.
Custom rules can be used in many scenarios. For example, you might create a rule that checks for memory leaks after each test. Or a rule that automatically retries failed tests. By creating custom rules, you can make your tests more powerful and flexible, without adding complexity.
Test Driven Development (TDD) with JUnit
Test-Driven Development: It means development by writing tests first. The idea is simple: You start by writing a test that describes the behavior you want. Then you write the code that makes the test pass. Finally, you refactor the code to improve its design keeping the tests green.
JUnit is great for TDD. You can write tests fast and then run them, keeping the TDD cycle really fast and efficient. That way, you will focus on the requirements and design of your code. You are guaranteed that your code will be testable, meaning it will have a much better design.
Advantages of using TDD are great in number. The code is better in quality as all code is covered by tests in TDD. The quantity of bugs will be low because tests catch mistakes up front. TDD improves the design, too, because it makes you think about how others will use your code. Embracing TDD will enable you to create more reliable and maintainable applications in Java.
Continuous Integration and JUnit
Think about how you can integrate more JUnit tests into your CI/CD. Why? That is so your code could be tested at any one time automatically. On each change, the tests would trigger. That will help catch bugs early. A bug caught earlier in the cycle is easier to fix.
It is fairly easy to set up JUnit tests for a CI tool like Jenkins or GitHub Actions. You may create a job at the Jenkins end which will actually run your JUnit tests every time some code has been pushed to the repository. All you have to do is to configure the job to pull code, fire the tests, and report results accordingly.. If a test fails, Jenkins notifies you immediately.
GitHub Actions works similarly. You set up a workflow that triggers on certain events, like a push to the main branch. The workflow includes a step to run your JUnit tests. If the tests fail, the workflow fails, giving you instant feedback.
Automated testing in the CI/CD process offers many benefits. It speeds up development by catching bugs early. It also ensures that your codebase remains stable. You can merge changes confidently, knowing that the tests have already checked for issues. This leads to faster, more reliable releases.
Cross-Browser Testing with JUnit and LambdaTest
Cross-browser compatibility is vital for Java applications. Users might access your application on different browsers. If it doesn’t work well on one of them, you could lose users. That’s why cross-browser testing is important.
JUnit is great for testing the logic of your code. But what about testing how it behaves in different browsers? That’s where LambdaTest comes in. LambdaTest allows you to run your JUnit tests across a wide range of browsers and devices. It’s like testing in a real-world environment, but from your development machine.
Integrating LambdaTest with JUnit is simple. You set up your JUnit tests as usual, but configure them to run on LambdaTest’s cloud. The tests execute on different browsers and devices, just as they would on a user’s machine. You get detailed results, showing how your application performed on each platform.
Using LambdaTest offers clear advantages. Its real device cloud enhances your testing capabilities. You can test on actual devices, not just emulators. This gives you more accurate results. You can identify and fix issues that might not appear in a standard testing environment.
Imagine running a JUnit test suite across Chrome, Firefox, Safari, and Edge. With LambdaTest, you can do this effortlessly. The platform handles the setup and execution. You focus on the results. This saves time and ensures your application works well for all users.
When using LambdaTest, there are best practices to keep in mind. Optimize your tests by focusing on critical paths. This reduces the time it takes to run tests across multiple platforms. Also, use LambdaTest’s parallel testing feature. It speeds up the process by running multiple tests at the same time.
Performance Testing with JUnit
It’s not about whether the code works or not, but about how well it performs under load.
Additional tools enable JUnit to do performance testing, too. One of these tools is JUnitPerf, which allows the execution of performance tests while running normal JUnit tests. JUnitPerf already allows the measurements of response times, throughput, and resource usage.
For example, you might write a test that measures exactly how long your application takes to respond to some request. JUnitPerf will then execute that test several times, simulating a heavy load of incoming requests. The results help you identify bottlenecks and optimize performance.
Best Practices for Writing Robust JUnit Tests
Writing effective JUnit tests is an art. To ensure your tests are robust, follow these key practices:
- Keep Tests Simple: Write tests that are easy to understand. Avoid complex logic in tests.
- Focus on One Thing: Each test should verify one aspect of your code. This makes it easier to identify what went wrong when a test fails.
- Use Descriptive Names: Test method names should describe what the test is checking. This makes it easier to understand the purpose of each test.
- Avoid Common Pitfalls: Don’t rely on external systems like databases or web services. Use mocks and stubs instead.
- Ensure Scalability: Write tests that can grow with your codebase. Avoid hardcoding values that might change.
Conclusion
In this blog, we explored advanced JUnit testing strategies. These strategies help make your Java applications more reliable. From parameterized tests to cross-browser testing with LambdaTest, each technique adds value.
Remember, testing is not a one-time task. It’s an ongoing process. By continuously learning and integrating new tools, like LambdaTest and stable tech stack, like selenium java you stay ahead. This ensures your applications remain robust, regardless of how they evolve. Keep testing, keep improving, and your code will always be ready for whatever comes next.