Android Automation: Continuous Testing Pipeline
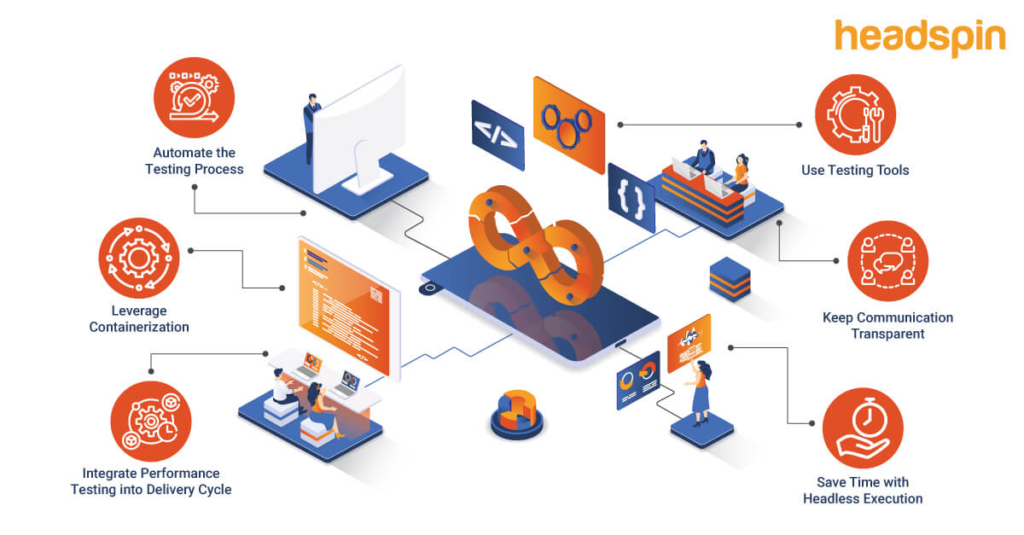
Currently, more and more users require fast applications that have almost no bugs and run on Android platforms, and therefore, the quality of Android applications has become crucial. Both CI and CD have radically transformed how teams release software, and integrating continuous testing into Android automation is a natural progression in delivering great user experiences.
In this article, we look into the preliminary need, integration, instruments, and procedure of Android automation into the CI/CD pipeline, which is helpful for both QA engineers and developers to provide quality apps.
The Importance of a Continuous Testing Pipeline
While Agile and DevOps are the leading models in the software development world today, continuous testing remains the only way of producing coherent Android products. Here’s why:
- Rapid Release Cycles: Automated testing enables the testing of every single commit that is written on the code so as to enhance faster and more secure releases.
- Enhanced Code Quality: Automated testing helps to avoid backsliding, code compliance with a previously set standard, and bug captures during the development phase.
- Device Fragmentation Challenges: Android devices have what can be described as a highly diverse market especially in terms of screen size, OS version, and overall hardware. This dispersed system’s permanency ensures compatibility by the constant testing of its components.
- User Experience Consistency: Preventive testing helps avoid problem occurrences during app usage, which makes the interaction easy and pleasant for users.
Key Components of a Continuous Testing Pipeline
The key components of a Continuous Testing Pipeline are:
- Source Code Management: A source code repository such as GitHub, GitLab, or Bitbucket serves as the foundation for collaboration and version control. Pipelines are triggered upon commits or pull requests.
- Build Automation: Tools like Gradle and Maven handle build automation by compiling code, resolving dependencies, and generating APKs.
- Automated Testing Frameworks: Frameworks like Espresso, JUnit, andAppium automate the execution of unit, integration, and UI tests.
- CI/CD Tools: Tools such as Jenkins, CircleCI, and GitHub Actions facilitate automated workflows for building, testing, and deploying Android apps.
- Device Testing Platforms: One must utilize Cloud services that provide access to real devices and emulators for comprehensive testing.
Setting Up a Continuous Testing Pipeline
Here’s a step-by-step guide on how to set up a CTP:
Step 1: Define Objectives and Scope
Start by identifying the primary goals of your pipeline:
– What kinds of tests will be automated? (e.g., unit, UI, performance)
– What device types, OS versions, and configurations should be covered?
– What performance metrics need monitoring?
Step 2: Choose Tools for Development and Testing
Select tools that align with your project requirements:
-Android Studio for development.
-Espresso is used for UI testing, and JUnit is used for unit testing.
-GitHub Actions or Jenkins are used for CI/CD automation.
Step 3: Automate the Build Process
UseGradle to simplify and automate builds. For example, you can configure Gradle scripts to generate debug and release builds:
“`groovy
android {
buildTypes {
debug {
debuggable true
}
release {
minifyEnabled true
proguardFiles getDefaultProguardFile(‘proguard-android-optimize.txt’), ‘proguard-rules.pro’
}
}
}
“`
Step 4: Write and Execute Test Scripts
Create automated test scripts tailored to your app.
– Use Espresso for UI testing.
– Use Mockito for mocking dependencies in unit tests.
Step 5: Integrate Testing with CI/CD
Configure your CI/CD platform to trigger automated tests with each code commit. For example:
– WithJenkins, configure a Jenkinsfile to automate testing:
“`groovy
pipeline {
stages {
stage(‘Build’) {
steps {
sh ‘./gradlew build’
}
}
stage(‘Test’) {
steps {
sh ‘./gradlew test’
}
}
}
}
“`
– WithGitHub Actions, use YAML workflows to integrate testing:
“`yaml
jobs:
build:
runs-on: ubuntu-latest
steps:
– name: Checkout code
uses: actions/checkout@v2
– name: Set up JDK
uses: actions/setup-java@v1
with:
java-version: ’11’
– name: Build with Gradle
run: ./gradlew build
– name: Run Tests
run: ./gradlew test
“`
Step 6: Device Testing
Ensure coverage across devices using emulators and cloud testing platforms:
– Set up Android emulators using AVD Manager in Android Studio.
– Use Firebase Test Lab to run tests on a range of real devices.
Types of Tests to Include
To build a robust pipeline, include a mix of automated test types:
- Unit Tests: Validate the most minor units of code in isolation. Use JUnit and Mockito for effective unit testing.
- Integration Tests: Ensure that components interact correctly. Tools like espresso andRobolectric can help test module integration.
- UI Tests: Automate user flows to verify the app’s interface. Espresso is a robust framework for this purpose.
- Performance Tests: Measure response times, memory usage, and CPU performance. Tools like Firebase Performance Monitoring are ideal for Android.
- Regression Tests: Regularly run tests to ensure new changes don’t break existing functionality.
Optimizing the Continuous Testing Pipeline
Let’s have a look at how to optimize the continuous testing pipeline:
Run Fast Tests First
Execute lightweight tests such as unit tests early in the pipeline to provide rapid feedback to developers.
Enable Parallel Test Execution
Use parallelization to run tests simultaneously on different devices or configurations, significantly reducing overall testing time.
Optimize Test Scripts
– Resolve flaky tests by addressing synchronization issues and dependencies.
– Use retries for transient failures.
Leverage Containerization
Use Docker to create standardized test environments, ensuring consistency across local and CI/CD environments.
Automate Reporting
Integrate tools like Allure Reports to generate detailed test execution reports for easier analysis and debugging.
Challenges and How to Overcome Them
Here are a few challenges and solutions on how to overcome them:
Device Fragmentation
Challenge: Testing across multiple devices and configurations is daunting.
Solution: Use cloud-based platforms like LambdaTest for comprehensive device coverage.
Test Flakiness
Challenge: Flaky tests lead to unreliable results.
Solution: Stabilize tests by using explicit waits and retry mechanisms.
Long Pipeline Execution Times
Challenge: Slow pipelines delay feedback.
Solution: Use parallel testing and split long-running tests into smaller modules.
Resource Constraints
Challenge: Limited resources hinder extensive testing.
Solution: Schedule tests during off-peak hours and leverage cloud solutions to scale on demand.
Best Practices for Continuous Testing
Continuous Testing (CT) is a critical component of modern software development and delivery pipelines. By embedding testing into every stage of the development lifecycle, teams can ensure higher quality, faster delivery, and seamless user experiences. To achieve these benefits, adopting the proper practices is essential. Here are a few of the best practices for implementing continuous testing effectively:
- Integrate Testing into CI/CD Pipelines
Automating testing as part of the CI/CD process enables the identification of problems early enough. This means that by running tests in the relevant steps in the pipeline, for instance, code commits, builds, and deployment, the teams can maintain quality. This method guarantees that potential production failures are quickly spotted and rectified, thus minimizing the effects of defects.
- Prioritize Automation
Automation is a cornerstone of efficient continuous testing. To execute key test cases daily, like regression, smoke, and functional tests, you can use Selenium, Appium, and Cypress. This also reduces the amount of work done by hand, the rate of errors, and the time spent testing. In large-scale or repetitive testing, automated testing has the added advantage of giving a consistent and standard result.
- Adopt Shift-Left Testing
Shift-left testing is characterized by the practice of commencing testing at a more advanced stage than conventional. It also implies engaging testers, who can look for defects during requirement analysis or design phase. This proactive approach helps avoid the likelihood of solving problems that emanate from expensive faulty software.
- Focus on Coverage
Comprehensive test coverage is essential for effective continuous testing. It includes unit, integration, system, and acceptance tests. Using tools that measure code coverage ensures that critical areas of the application are thoroughly tested. By addressing coverage gaps, teams can deliver more reliable and robust applications.
- Ensure Cross-Platform and Cross-Browser Testing
It is important that your website works for many platforms and can be accessed in multiple browsers today. By leveraging platforms such as LambdaTest, a group of developers can test their applications on different devices, OS, and browser environments. This helps provide a consistent experience once the users use the website in different environments.
Cross-browser testing is an important function that any developer or tester cannot afford to overlook, which is why LambdaTest is a prominent cloud-based solution. LambdaTest can support more than 3000 browser & OS combinations and help the teams verify the proper functioning of their web applications in many environments.
This service provides live browser testing and has an intelligent testing feature. Therefore, it is suitable for any company that wants to ensure quality. Parallel testing can also be performed on the platform, which saves lots of time for testing and, hence, overall development time.
Another impressive feature offered by LambdaTest is that it allows users to create real conditions, such as different screen sizes, geo-locations, and network conditions. For developers testing mobile applications on macOS, LambdaTest also provides seamless integration with an Android emulator Mac, ensuring that mobile app testing is as efficient and accurate as web app testing. This guarantees that your application will respond well, no matter the circumstances surrounding it.
It also has built-in compatibility with tools such as Jenkins and GitHub and seamless integration with other tools such as Jira. This makes the application suitable for DevOps. Further, implementing application performance monitoring allows specific, accurate reporting and debugging for practitioners, catering to teams who focus on detecting and fixing problems, such as agility, bettering the end consumer experience, and value realization.
- Monitor Test Metrics
Test metrics make it easier to know whether your testing strategy is working. They provide obvious measures of improvement, like defect density, test coverage, and execution time. Real-time dashboards contain information that can be used to make decisions because numbers indicate that teams require optimization.
- Embrace Continuous Feedback Loop
Continuous testing thrives on actionable feedback at every stage of the development cycle. By including feedback details, developers, testers, and other working entities can encourage cross-communication. This may include bug tracking, test reports, and real-time notifications. Software such as Jira, Slack, etc., is good to incorporate in the process, hence enhancing the transparency needed. It ensures that issues are promptly addressed, leading to a more refined and reliable product.
- Regularly Update Test Suites
As the application evolves, so should your test cases. It is recommended that you go through your test suites with the necessary frequency and add changes corresponding to new features and bug fixes. Old-fashioned tests can sometimes give you wrong and negative outcomes that will greatly affect the effectiveness of your testing. Conduct periodic audits to ensure that automated and manual tests remain relevant and efficient, saving time and resources in the long run.
When effectively applied in an organization, these practices will go a long way toward laying the groundwork for sustainable continuous testing, which will not only enhance the quality of the software delivered but also improve the delivery speed and return value to end users.
Future Trends in Android Automation
Let’s have a look at some of the future trends in Android automation:
AI-Powered Testing:
AI tools likeTest.ai predict app behaviors and optimize test execution.
Machine learning helps create innovative test cases and detect patterns.
Mobile DevOps Expansion:
DevOps practices explicitly tailored for mobile will see greater adoption, with tighter integration between testing, development, and deployment.
5G Testing:
The advent of 5G will necessitate testing for low latency and high-speed scenarios.
Enhanced Security Testing:
Continuous pipelines will incorporate automated security testing to detect vulnerabilities proactively.
Conclusion
Testing within the Android automation process has become mandatory, and regular testing must be incorporated into the process if application developers are to provide the best products within the shortest time possible in the current market. When teams use the right tools, solid processes and practices in place, and other techniques, feedback will be fast, code quality will not suffer, and users can enjoy applications on many devices with multiple configurations.
Thus, an adaptation of innovations like AI testing and cloud-based device platforms will make mobile ecosystems more scalable for Android ecosystems. Through a proactive approach of continuous testing, organizations can solve the challenges, increase the speed of releasing new features, and satisfy the increasing demands of modern users. It not only leads to significant and high-quality products but also retains the confidence and satisfaction of the user to use the product in the long term.